Check Nested Relations with One Method Call
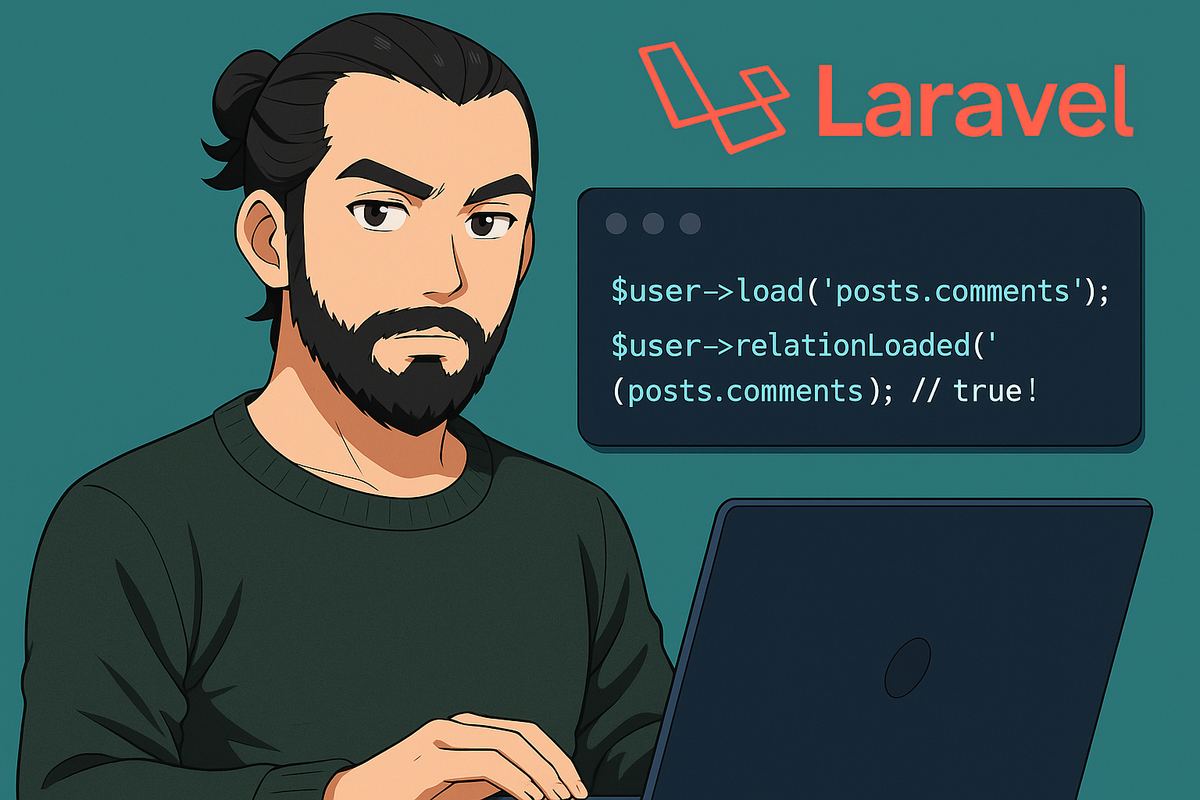
When working with Eloquent models in Laravel, relationship management is a cornerstone of efficient database interaction. We've always had powerful tools for loading nested relationships with elegant dot notation, but until now, there was a surprising inconsistency in how we could verify those relationships were loaded.
The Relationship Gap
Laravel has long allowed developers to eager load nested relationships using dot notation. This syntax is intuitive and expressive:
$user->load('posts.comments');
With a single line, you can load a user's posts and all comments associated with those posts. This prevents the dreaded N+1 query problem and keeps your application running efficiently.
However, before Laravel 12.10, when you wanted to check if these relationships were loaded, the relationLoaded()
method only worked for single-level relationships:
// Before Laravel 12.10
$user->load('posts.comments');
$user->relationLoaded('posts'); // true
$user->relationLoaded('posts.comments'); // false
This inconsistency meant developers had to write additional code to verify nested relationships, often resorting to workarounds like:
// Workaround for checking nested relations
if ($user->relationLoaded('posts') && $user->posts->first()?->relationLoaded('comments')) {
// Posts and their comments are loaded
}
The Enhancement
Thanks to a contribution from Mahesh Perera in Laravel 12.10, the relationLoaded()
method now supports the same dot notation we use for loading relationships:
// After Laravel 12.10
$user->load('posts.comments');
$user->relationLoaded('posts'); // true
$user->relationLoaded('posts.comments'); // true
This seemingly small change eliminates the inconsistency between how we load and verify relationships, making our code more intuitive and less prone to errors.
Real-World Example
Let's see how this enhancement simplifies a common pattern in Laravel applications. Imagine you're building a blog with a controller method that displays a post with its comments and author information.
With the enhanced relationLoaded()
method, your code becomes more straightforward and efficient:
public function show(Post $post)
{
// Load only what we need
$relations = [];
if (!$post->relationLoaded('author')) {
$relations[] = 'author';
}
if (!$post->relationLoaded('comments.author')) {
$relations[] = 'comments.author';
}
if (!empty($relations)) {
$post->load($relations);
}
return view('posts.show', compact('post'));
}
This approach prevents unnecessary database queries by only loading relationships that aren't already loaded, improving your application's performance.
Stay Updated with More Laravel Content
Enjoyed this article? There's plenty more where that came from! Subscribe to our channels to stay updated with the latest Laravel tips, tricks, and best practices:
- Follow us on Twitter @harrisrafto
- Join us on Bluesky @harrisrafto.eu
- Subscribe to our YouTube channel