Customizing Error Pages in Laravel
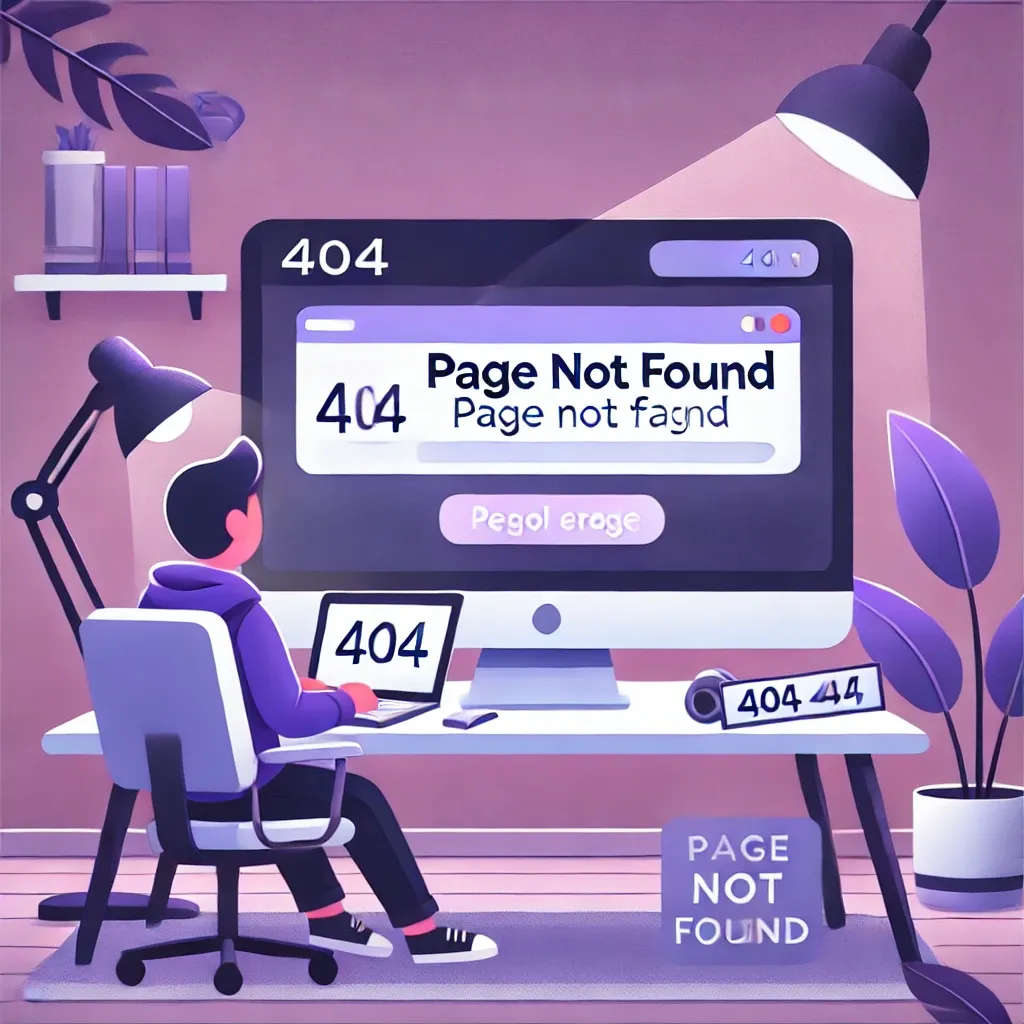
Learn how to create and customize error pages for different HTTP status codes in Laravel, making your application's error handling both user-friendly and professional.
Basic Error Page Creation
Create custom error pages by adding Blade templates in the errors directory:
// resources/views/errors/404.blade.php
<h2>{{ $exception->getMessage() }}</h2>
Publishing Default Templates
php artisan vendor:publish --tag=laravel-errors
Real-World Example
Here's how to create a comprehensive error handling system:
// resources/views/errors/layout.blade.php
<!DOCTYPE html>
<html>
<head>
<title>@yield('title', 'Error')</title>
<style>
.error-container {
text-align: center;
padding: 40px;
}
.error-code {
font-size: 72px;
color: #e74c3c;
}
</style>
</head>
<body>
<div class="error-container">
@yield('content')
@if(app()->environment('local'))
<div class="debug-info">
{{ $exception->getMessage() }}
</div>
@endif
</div>
</body>
</html>
// resources/views/errors/404.blade.php
@extends('errors.layout')
@section('title', '404 Not Found')
@section('content')
<div class="error-code">404</div>
<h1>Page Not Found</h1>
<p>The page you're looking for doesn't exist.</p>
<a href="{{ url('/') }}">Return Home</a>
@endsection
// resources/views/errors/500.blade.php
@extends('errors.layout')
@section('title', '500 Server Error')
@section('content')
<div class="error-code">500</div>
<h1>Server Error</h1>
<p>We are experiencing some technical difficulties.</p>
<p>Please try again later.</p>
@endsection
// resources/views/errors/4xx.blade.php
@extends('errors.layout')
@section('title', 'Client Error')
@section('content')
<div class="error-code">{{ $exception->getStatusCode() }}</div>
<h1>Oops! Something went wrong</h1>
<p>{{ $exception->getMessage() ?: 'An error occurred while processing your request.' }}</p>
@endsection
The setup provides a consistent error page layout while handling different error scenarios appropriately.
If this guide was helpful to you, subscribe to my daily newsletter and give me a follow on X/Twitter and Bluesky. It helps a lot!