Enhanced Time Testing in Laravel with Improved Freeze Methods
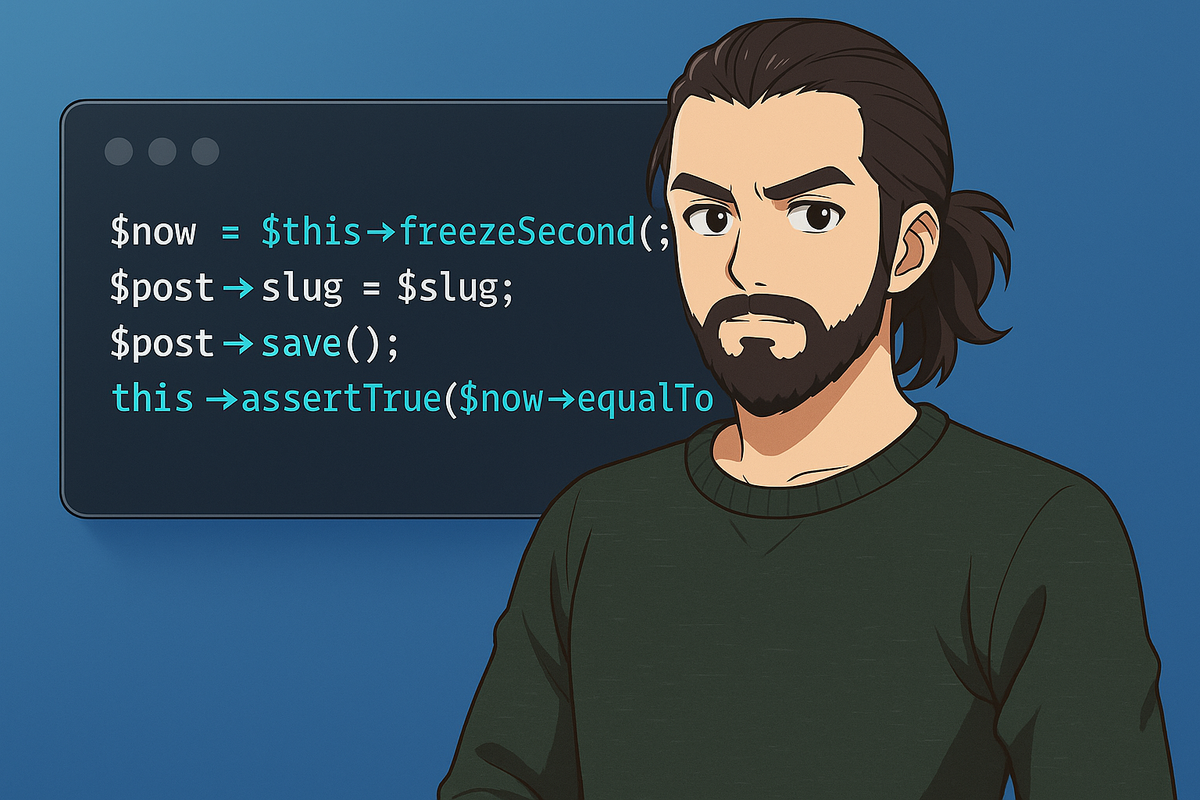
Laravel improves testing convenience with enhanced freezeTime() and freezeSecond() methods that return the frozen timestamp for easier test assertions.
When testing time-sensitive functionality, you often need to freeze time and then access that exact timestamp for assertions. The updated freeze methods now return the frozen time directly, eliminating the need for manual Carbon manipulations and making your tests cleaner.
Let's see how it works:
// Before
$now = now();
Carbon::setTestNow($now);
$post->slug = $slug;
$post->save();
$post->refresh();
$this->assertTrue($now->equalTo($post->updated_at));
// After
$now = $this->freezeSecond();
$post->slug = $slug;
$post->save();
$post->refresh();
$this->assertTrue($now->equalTo($post->updated_at));
Real-World Example
Here's how you might use these enhanced methods in your test suite:
class OrderProcessingTest extends TestCase
{
public function test_order_processing_timestamps()
{
$processedAt = $this->freezeTime();
$order = Order::factory()->create();
$order->process();
$this->assertEquals($processedAt, $order->processed_at);
}
public function test_subscription_renewal()
{
$renewedAt = $this->freezeSecond();
$subscription = Subscription::factory()->create([
'expires_at' => now()->subDay()
]);
$subscription->renew();
$this->assertTrue($renewedAt->equalTo($subscription->renewed_at));
$this->assertTrue($renewedAt->addMonth()->equalTo($subscription->expires_at));
}
}
These enhancements provide smoother testing experience by reducing boilerplate code and eliminating common time-related test gotchas. You get the frozen timestamp directly, making your assertions more straightforward and maintainable.
Stay Updated with More Laravel Tips
Enjoyed this article? There's plenty more where that came from! Subscribe to our channels to stay updated with the latest Laravel tips, tricks, and best practices:
- Follow us on Twitter @harrisrafto
- Join us on Bluesky @harrisrafto.eu
- Subscribe to our YouTube channel harrisrafto