Track Job Lifecycles with Laravel's Enhanced Queue Payloads
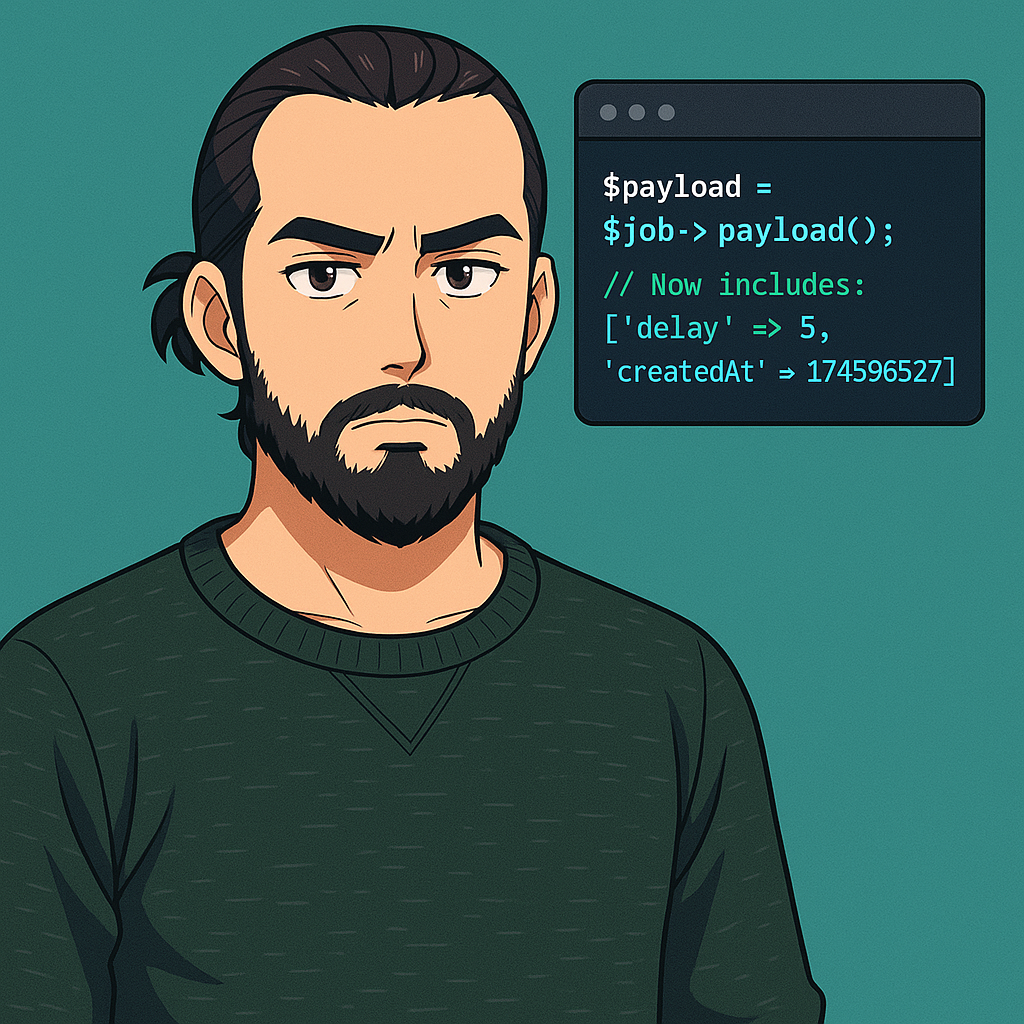
When working with Laravel's queue system, understanding the full lifecycle of a job is crucial for debugging, performance optimization, and system reliability. Until now, there's been a subtle but important gap in the information available about queued jobs: the exact creation time and delay settings weren't directly accessible in the job payload.
The Enhanced Job Payload
In the latest update, Laravel now includes two additional pieces of information in every job payload:
createdAt
- A timestamp indicating exactly when the job payload was createddelay
- The optional delay in seconds before the job should be processed
Here's how you can access this information:
$job->payload();
/*
Now includes: ['delay' => 5, 'createdAt' => 1745965273]
*/
This seemingly small addition provides crucial context that was previously difficult to obtain programmatically.
Real-World Example
Let's see how these new payload fields enable better monitoring and debugging in a real application:
namespace App\Jobs;
use Illuminate\Bus\Queueable;
use Illuminate\Contracts\Queue\ShouldQueue;
use Illuminate\Foundation\Bus\Dispatchable;
use Illuminate\Queue\InteractsWithQueue;
use Illuminate\Queue\SerializesModels;
use Illuminate\Support\Carbon;
class ProcessOrderJob implements ShouldQueue
{
use Dispatchable, InteractsWithQueue, Queueable, SerializesModels;
public function __construct(
public int $orderId
) {}
public function handle()
{
// Get the job payload with the new information
$payload = $this->job->payload();
// Access the creation timestamp and convert to a Carbon instance
$createdAt = Carbon::createFromTimestamp($payload['createdAt']);
// Calculate how long the job was in the queue
$queueTime = now()->diffInSeconds($createdAt);
// Get the intended delay
$intendedDelay = $payload['delay'] ?? 0;
// Log queue processing time information
logger()->info("Processing Order #{$this->orderId}", [
'created_at' => $createdAt->toDateTimeString(),
'queue_time' => $queueTime,
'intended_delay' => $intendedDelay,
'actual_delay' => $queueTime - $intendedDelay,
]);
// Proceed with order processing...
}
}
Now, when dispatching this job with a delay:
ProcessOrderJob::dispatch($order->id)->delay(now()->addMinutes(5));
Your logs will contain detailed timing information that helps you understand exactly how your queue is performing.
Stay Updated with More Laravel Tips
Enjoyed this article? There's plenty more where that came from! Subscribe to our channels to stay updated with the latest Laravel tips, tricks, and best practices:
- Follow us on Twitter @harrisrafto
- Join us on Bluesky @harrisrafto.eu
- Subscribe to our YouTube channel harrisrafto