Type-Safe Array Access: Laravel's New Array Typed Getters
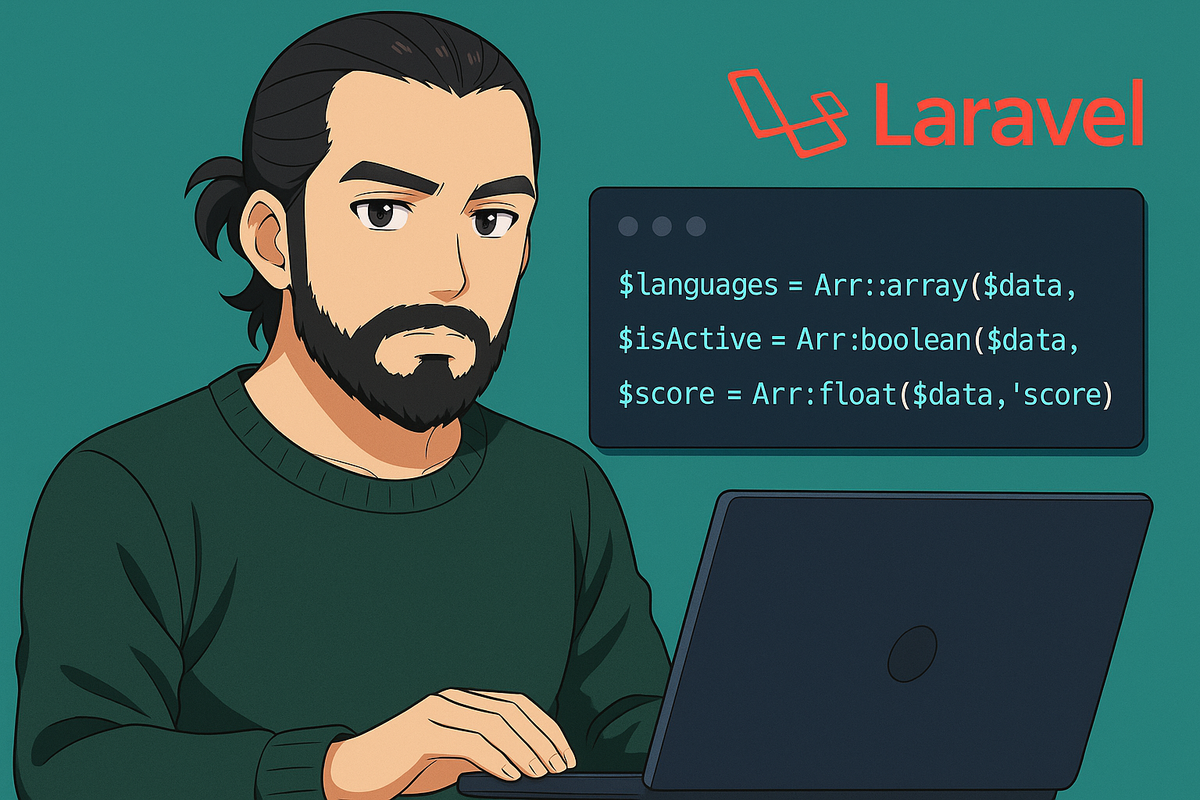
Arrays are fundamental to PHP development, but they can be a source of subtle bugs when you expect one type but get another. How many times have you written code like if (!empty($data['settings']) && is_array($data['settings']))
to ensure your data is of the right type before using it? Laravel's new typed getter helpers for the Arr
class eliminate this boilerplate while making your code both more concise and more robust.
Understanding Typed Getters
Laravel's Arr
class has been enhanced with typed getter methods that attempt to retrieve a value from an array and verify it matches the expected type. If the value doesn't match, it throws an InvalidArgumentException
, protecting you from unexpected data formats.
The available typed getters include:
use Illuminate\Support\Arr;
// Basic array example
$array = [
'name' => 'Joe',
'active' => true,
'score' => 85.5,
'visits' => 42,
'languages' => ['PHP', 'Ruby']
];
// Type-specific retrievals
$languages = Arr::array($array, 'languages'); // ['PHP', 'Ruby']
$isActive = Arr::boolean($array, 'active'); // true
$score = Arr::float($array, 'score'); // 85.5
$visits = Arr::integer($array, 'visits'); // 42
$name = Arr::string($array, 'name'); // 'Joe'
When the types don't match, the methods throw helpful exceptions:
// This will throw an InvalidArgumentException
$value = Arr::array($array, 'name'); // 'name' is a string, not an array
Real-World Example
Let's see how typed getters improve a typical configuration-processing scenario:
use Illuminate\Support\Arr;
class PaymentGateway
{
protected array $config;
public function __construct(array $config)
{
$this->config = $config;
}
public function processPayment(float $amount): bool
{
// Before: verbose type checking
if (!isset($this->config['api_key']) || !is_string($this->config['api_key'])) {
throw new \Exception('API key is required and must be a string');
}
if (isset($this->config['debug']) && !is_bool($this->config['debug'])) {
throw new \Exception('Debug flag must be a boolean value');
}
if (isset($this->config['allowed_currencies']) && !is_array($this->config['allowed_currencies'])) {
throw new \Exception('Allowed currencies must be an array');
}
// Process payment...
// After: concise, expressive type checking
try {
$apiKey = Arr::string($this->config, 'api_key');
$debug = Arr::boolean($this->config, 'debug', false); // With default
$allowedCurrencies = Arr::array($this->config, 'allowed_currencies', []); // With default
// Process payment...
} catch (\InvalidArgumentException $e) {
throw new \Exception('Invalid payment configuration: ' . $e->getMessage());
}
}
}
Notice how the typed getters reduce boilerplate while making the intent clearer. The code now focuses on what we need rather than tedious validation.
Stay Updated with More Laravel Tips
Enjoyed this article? There's plenty more where that came from! Subscribe to our channels to stay updated with the latest Laravel tips, tricks, and best practices:
- Follow us on Twitter @harrisrafto
- Join us on Bluesky @harrisrafto.eu
- Subscribe to our YouTube channel harrisrafto